力扣-102.二叉树的层序遍历
给你二叉树的根节点 root
,返回其节点值的 层序遍历 。 (即逐层地,从左到右访问所有节点)。
示例 1:
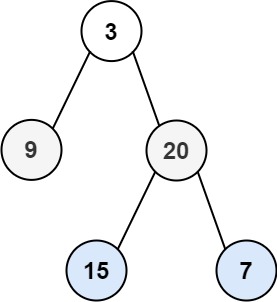
1 2
| 输入:root = [3,9,20,null,null,15,7] 输出:[[3],[9,20],[15,7]]
|
示例 2:
示例 3:
代码实现如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| /** * Definition for a binary tree node. * function TreeNode(val, left, right) { * this.val = (val===undefined ? 0 : val) * this.left = (left===undefined ? null : left) * this.right = (right===undefined ? null : right) * } */ /** * @param {TreeNode} root * @return {number[][]} */ var levelOrder = function(root) { let queue = [root] let res = [] if(root && root.val!=null)//坑:最开始写root&&root.val当root.val=0时push不进去 res.push([root.val]) let curnum = 1 let nextnum = 0 let tmp = [] while(queue[0]){ tmp = tmp.length>0 ? tmp : [] if(queue[0].left){ queue.push(queue[0].left) tmp.push(queue[0].left.val) nextnum++ } if(queue[0].right){ queue.push(queue[0].right) tmp.push(queue[0].right.val) nextnum++ } queue.shift() curnum-- if(curnum === 0){ if(tmp.length>0) res.push(tmp) tmp = [] curnum = nextnum nextnum = 0 } } return res };
|
解题思路:
用队列实现二叉树的广度优先遍历
用两个变量分别记录两层节点的数量,curnum记录当前层中位于队列中节点的数量,nextnum记录下一层中位于队列中节点的数量